Using Design Patterns in PHP
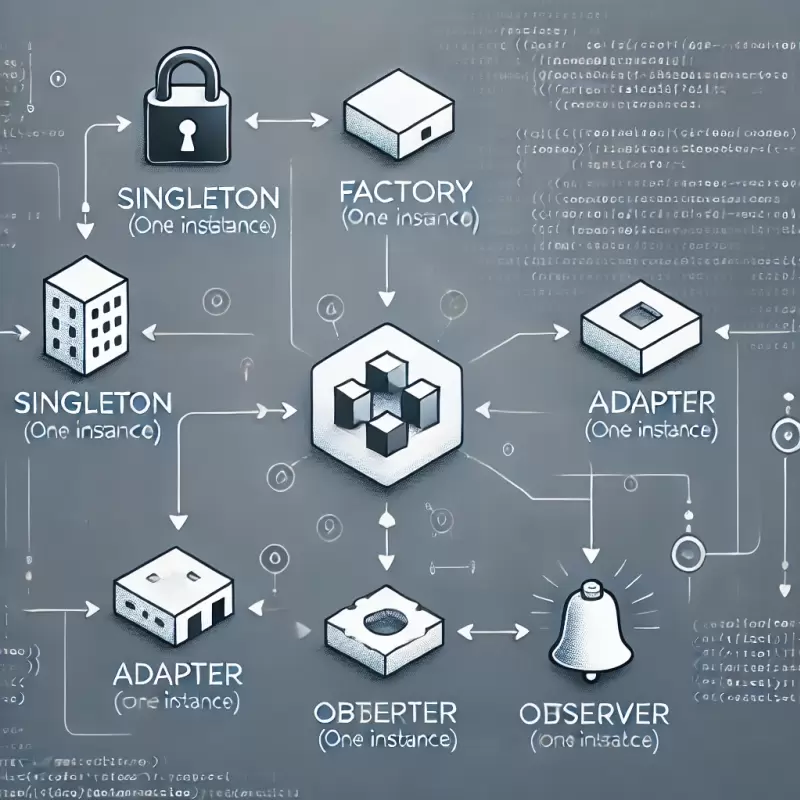
Design Patterns are proven solutions to common problems that arise in software development. They provide best practices for structuring and designing systems in an efficient manner. Design Patterns can be categorized into three main groups: Creational Patterns, Structural Patterns, and Behavioral Patterns. This article will explain examples of these patterns in PHP.
1. Creational Patterns
Creational Patterns focus on object creation, providing a way to instantiate objects more efficiently without creating them directly. Notable examples include
1.1 Singleton Pattern
The Singleton Pattern ensures that only one instance of a class is created and used throughout the application, often used for managing database connections.
class Singleton {
private static $instance;
private function __construct() {}
public static function getInstance() {
if (self::$instance === null) {
self::$instance = new Singleton();
}
return self::$instance;
}
}
1.2 Factory Pattern
The Factory Pattern allows for creating objects without specifying the exact class of the object to be created.
class ProductFactory {
public static function create($type) {
if ($type === 'A') {
return new ProductA();
} elseif ($type === 'B') {
return new ProductB();
}
throw new Exception("Invalid product type");
}
}
1.3 Abstract Factory Pattern
The Abstract Factory Pattern is an extension of the Factory Pattern. It is used to create objects that belong to families of related objects.
interface GUIFactory {
public function createButton();
}
class WinFactory implements GUIFactory {
public function createButton() {
return new WinButton();
}
}
class MacFactory implements GUIFactory {
public function createButton() {
return new MacButton();
}
}
1.4 Builder Pattern
The Builder Pattern separates the construction of complex objects into smaller, manageable steps, making it easier to build objects flexibly.
class Car {
private $engine;
private $wheels;
public function setEngine($engine) {
$this->engine = $engine;
}
public function setWheels($wheels) {
$this->wheels = $wheels;
}
}
class CarBuilder {
private $car;
public function __construct() {
$this->car = new Car();
}
public function addEngine($engine) {
$this->car->setEngine($engine);
}
public function addWheels($wheels) {
$this->car->setWheels($wheels);
}
public function build() {
return $this->car;
}
}
1.5 Prototype Pattern
The Prototype Pattern creates new objects by cloning an existing object.
class Prototype {
public $name;
public function __clone() {
// Clone the object
}
}
$prototype = new Prototype();
$clone = clone $prototype;
2. Structural Patterns
Structural Patterns focus on organizing classes and objects and defining how they interact with each other. Key examples include
2.1 Adapter Pattern
The Adapter Pattern allows classes with incompatible interfaces to work together by adapting the interface of one class to match another.
interface MediaPlayer {
public function play($filename);
}
class MP3Player implements MediaPlayer {
public function play($filename) {
echo "Playing MP3 file: " . $filename;
}
}
class MP4Adapter implements MediaPlayer {
private $mp4Player;
public function __construct(MP4Player $mp4Player) {
$this->mp4Player = $mp4Player;
}
public function play($filename) {
$this->mp4Player->playMP4($filename);
}
}
2.2 Facade Pattern
The Facade Pattern hides the complexity of a system by providing a simplified interface for users to interact with.
class CPU {
public function start() {
echo "CPU started.";
}
}
class Memory {
public function load() {
echo "Memory loaded.";
}
}
class HardDrive {
public function read() {
echo "Hard drive reading data.";
}
}
class ComputerFacade {
private $cpu;
private $memory;
private $hardDrive;
public function __construct() {
$this->cpu = new CPU();
$this->memory = new Memory();
$this->hardDrive = new HardDrive();
}
public function start() {
$this->cpu->start();
$this->memory->load();
$this->hardDrive->read();
}
}
2.3 Composite Pattern
The Composite Pattern allows you to treat individual objects and compositions of objects uniformly by organizing them in a tree structure.
interface Component {
public function operation();
}
class Leaf implements Component {
public function operation() {
echo "Leaf operation\n";
}
}
class Composite implements Component {
private $children = [];
public function add(Component $component) {
$this->children[] = $component;
}
public function operation() {
foreach ($children as $child) {
$child->operation();
}
}
}
2.4 Decorator Pattern
The Decorator Pattern allows for extending the behavior of objects dynamically without modifying their code.
interface Coffee {
public function cost();
}
class SimpleCoffee implements Coffee {
public function cost() {
return 50;
}
}
class MilkDecorator implements Coffee {
protected $coffee;
public function __construct(Coffee $coffee) {
$this->coffee = $coffee;
}
public function cost() {
return $this->coffee->cost() + 20;
}
}
2.5 Proxy Pattern
The Proxy Pattern provides a substitute for another object to control access to it, such as for caching or logging.
interface Image {
public function display();
}
class RealImage implements Image {
private $filename;
public function __construct($filename) {
$this->filename = $filename;
$this->loadFromDisk();
}
private function loadFromDisk() {
echo "Loading " . $this->filename . "\n";
}
public function display() {
echo "Displaying " . $this->filename . "\n";
}
}
class ProxyImage implements Image {
private $realImage;
private $filename;
public function __construct($filename) {
$this->filename = $filename;
}
public function display() {
if ($this->realImage === null) {
$this->realImage = new RealImage($this->filename);
}
$this->realImage->display();
}
}
3. Behavioral Patterns
Behavioral Patterns focus on communication and interaction between objects. Examples include
3.1 Observer Pattern
The Observer Pattern allows an object to notify other objects about changes in its state.
class Subject {
private $observers = [];
public function addObserver($observer) {
$this->observers[] = $observer;
}
public function notifyObservers() {
foreach ($this->observers as $observer) {
$observer->update();
}
}
}
class ConcreteObserver {
public function update() {
echo "Observer notified!\n";
}
}
3.2 Strategy Pattern
The Strategy Pattern allows you to change the behavior of an object at runtime by selecting different strategies.
interface Strategy {
public function execute($a, $b);
}
class AddStrategy implements Strategy {
public function execute($a, $b) {
return $a + $b;
}
}
3.3 Command Pattern
The Command Pattern separates the request for an action from the object that performs the action, making it easier to undo or store commands.
interface Command {
public function execute();
}
class Light {
public function turnOn() {
echo "Light is on\n";
}
}
class TurnOnCommand implements Command {
private $light;
public function __construct(Light $light) {
$this->light = $light;
}
public function execute() {
$this->light->turnOn();
}
}
3.4 Chain of Responsibility Pattern
The Chain of Responsibility Pattern passes requests along a chain of handlers that can choose to process the request or pass it on.
abstract class Handler {
protected $nextHandler;
public function setNext(Handler $handler) {
$this->nextHandler = $handler;
return $handler;
}
public function handle($request) {
if ($this->nextHandler) {
$this->nextHandler->handle($request);
}
}
}
3.5 Mediator Pattern
The Mediator Pattern provides an intermediary object to handle communication between multiple objects, allowing them to avoid direct communication.
interface Mediator {
public function send($message, Colleague $colleague);
}
Conclusion
Using Design Patterns in PHP helps make your code more structured, flexible, and easier to maintain. Choosing the right pattern for your project's structure will enhance development efficiency and future scalability.
By studying and understanding various Design Patterns, you'll be able to write more efficient and systematic code, which is crucial for solving complex problems.